자바스크립트, 제이쿼리 활용하여 달력 만들기
윤년 적용
오늘 날짜 색 다르게
이전달, 다음달 버튼 구현하기
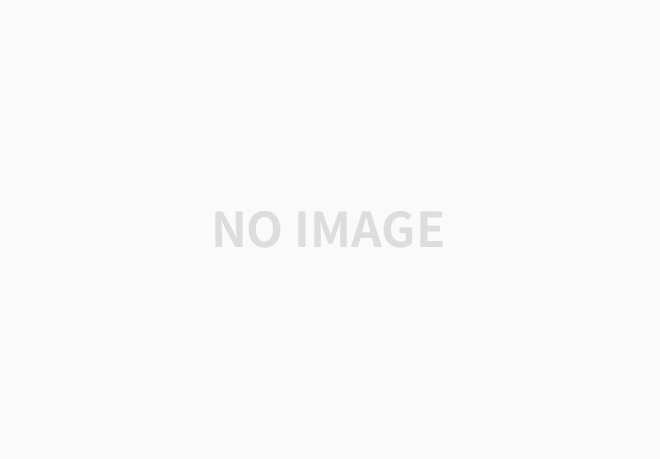
html code
<table id="calendar">
<thead>
<tr>
<th><input name="preMon" type="button" value="<"></th>
<th colspan="5" class="year_mon"></th>
<th><input name="nextMon" type="button" value=">"></th>
</tr>
<tr>
<th>일</th>
<th>월</th>
<th>화</th>
<th>수</th>
<th>목</th>
<th>금</th>
<th>토</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
javascript code
<script>
$(function(){
var today = new Date();
var date = new Date();
$("input[name=preMon]").click(function() { // 이전달
$("#calendar > tbody > td").remove();
$("#calendar > tbody > tr").remove();
today = new Date ( today.getFullYear(), today.getMonth()-1, today.getDate());
buildCalendar();
})
$("input[name=nextMon]").click(function(){ //다음달
$("#calendar > tbody > td").remove();
$("#calendar > tbody > tr").remove();
today = new Date ( today.getFullYear(), today.getMonth()+1, today.getDate());
buildCalendar();
})
function buildCalendar() {
nowYear = today.getFullYear();
nowMonth = today.getMonth();
firstDate = new Date(nowYear,nowMonth,1).getDate();
firstDay = new Date(nowYear,nowMonth,1).getDay(); //1st의 요일
lastDate = new Date(nowYear,nowMonth+1,0).getDate();
if((nowYear%4===0 && nowYear % 100 !==0) || nowYear%400===0) { //윤년 적용
lastDate[1]=29;
}
$(".year_mon").text(nowYear+"년 "+(nowMonth+1)+"월");
for (i=0; i<firstDay; i++) { //첫번째 줄 빈칸
$("#calendar tbody:last").append("<td></td>");
}
for (i=1; i <=lastDate; i++){ // 날짜 채우기
plusDate = new Date(nowYear,nowMonth,i).getDay();
if (plusDate==0) {
$("#calendar tbody:last").append("<tr></tr>");
}
$("#calendar tbody:last").append("<td class='date'>"+ i +"</td>");
}
if($("#calendar > tbody > td").length%7!=0) { //마지막 줄 빈칸
for(i=1; i<= $("#calendar > tbody > td").length%7; i++) {
$("#calendar tbody:last").append("<td></td>");
}
}
$(".date").each(function(index){ // 오늘 날짜 표시
if(nowYear==date.getFullYear() && nowMonth==date.getMonth() && $(".date").eq(index).text()==date.getDate()) {
$(".date").eq(index).addClass('colToday');
}
})
}
buildCalendar();
})
</script>
css code
<style>
table {
clear: both;
}
th {
height: 50px;
width: 70px;
background-color: orange;
}
td {
text-align: center;
height: 50px;
width: 70px;
background-color: #FFFDC5;
}
input {
height: 50px;
width: 70px;
border: none;
background-color: orange;
font-size: 30px;
}
.year_mon{
font-size: 25px;
}
.colToday{
background-color: #FFA07A;
}
</style>
전체 코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<title>calendar</title>
<style>
table {
clear: both;
}
th {
height: 50px;
width: 70px;
background-color: orange;
}
td {
text-align: center;
height: 50px;
width: 70px;
background-color: #FFFDC5;
}
input {
height: 50px;
width: 70px;
border: none;
background-color: orange;
font-size: 30px;
}
.year_mon{
font-size: 25px;
}
.colToday{
background-color: #FFA07A;
}
</style>
<script>
$(function(){
var today = new Date();
var date = new Date();
$("input[name=preMon]").click(function() { // 이전달
$("#calendar > tbody > td").remove();
$("#calendar > tbody > tr").remove();
today = new Date ( today.getFullYear(), today.getMonth()-1, today.getDate());
buildCalendar();
})
$("input[name=nextMon]").click(function(){ //다음달
$("#calendar > tbody > td").remove();
$("#calendar > tbody > tr").remove();
today = new Date ( today.getFullYear(), today.getMonth()+1, today.getDate());
buildCalendar();
})
function buildCalendar() {
nowYear = today.getFullYear();
nowMonth = today.getMonth();
firstDate = new Date(nowYear,nowMonth,1).getDate();
firstDay = new Date(nowYear,nowMonth,1).getDay(); //1st의 요일
lastDate = new Date(nowYear,nowMonth+1,0).getDate();
if((nowYear%4===0 && nowYear % 100 !==0) || nowYear%400===0) { //윤년 적용
lastDate[1]=29;
}
$(".year_mon").text(nowYear+"년 "+(nowMonth+1)+"월");
for (i=0; i<firstDay; i++) { //첫번째 줄 빈칸
$("#calendar tbody:last").append("<td></td>");
}
for (i=1; i <=lastDate; i++){ // 날짜 채우기
plusDate = new Date(nowYear,nowMonth,i).getDay();
if (plusDate==0) {
$("#calendar tbody:last").append("<tr></tr>");
}
$("#calendar tbody:last").append("<td class='date'>"+ i +"</td>");
}
if($("#calendar > tbody > td").length%7!=0) { //마지막 줄 빈칸
for(i=1; i<= $("#calendar > tbody > td").length%7; i++) {
$("#calendar tbody:last").append("<td></td>");
}
}
$(".date").each(function(index){ // 오늘 날짜 표시
if(nowYear==date.getFullYear() && nowMonth==date.getMonth() && $(".date").eq(index).text()==date.getDate()) {
$(".date").eq(index).addClass('colToday');
}
})
}
buildCalendar();
})
</script>
</head>
<body>
<table id="calendar">
<thead>
<tr>
<th><input name="preMon" type="button" value="<"></th>
<th colspan="5" class="year_mon"></th>
<th><input name="nextMon" type="button" value=">"></th>
</tr>
<tr>
<th>일</th>
<th>월</th>
<th>화</th>
<th>수</th>
<th>목</th>
<th>금</th>
<th>토</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</body>
</html>
공부하기 시작한 초반엔 만들지도 못했던 달력,,,, 그 떄 한 삼 일 동안 만들다가 포기했었다 ㅎㅎ
공부하기 두 달차 제이쿼리를 활용해 만들었더니 손쉽게(?) 완성 그래도 반나절 걸리긴 했지만
그래도 했다는 것에 다행으로 생각하며 뿌듯하게 마무리!
'공부 > JS , HTML , CSS' 카테고리의 다른 글
[javascript/jqeury] 세로메뉴, 메뉴 클릭시 서브메뉴 열기 (0) | 2021.05.03 |
---|---|
[javascript] table tr 복사(clone()), tr의 td삭제하기, 버튼 클릭 시 테이블 합치기(rowspan), 삭제버튼, class 이름바꿔서 추가하기 (2) | 2021.03.04 |
[javascript] select 옵션 선택마다 다른 사진 출력 (0) | 2021.02.28 |
[javascript] 사칙연산 계산기 만들기 ( javascript calculator) (0) | 2021.02.28 |